Monitor & Alert
Monitor & Alert
Webhook signature & security
To make your webhooks secure, you can verify that they originated from Tokenview by generating a HMAC SHA-256 hash code using your unique webhook signing key.
Find your signing key
Navigate to the setting page in your dashboard. Next, Click "Get": the Signing key is displayed and can be copied for use.
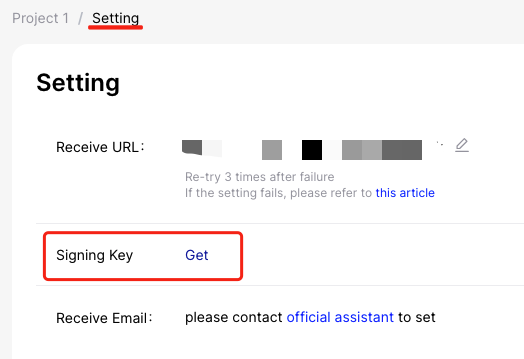
Validate the signature received
Every outbound request contains a hashed authentication signature in the header. It's computed by concatenating your signing key and request body. Then generates a hash using the HMAC SHA256 hash algorithm.
To verify the signature came from Tokenview, you generate the HMAC SHA256 hash and compare it with the signature received.
Example request header
Content-Type: application/json;charset=UTF-8
X-Tokenview-Signature: your-hashed-signature
Example signature validation (python)
import hmac
import hashlib
# abcde is the content to be encrypted, which is the body of the webhook message
data=bytes('abcde','utf-8')
key=bytes('signkey string','utf-8')
# digest is signature, which is the value of X-Tokenview-Signature
digest=hmac.new(key,data,digestmod=hashlib.sha256).hexdigest()
print(digest)
Set webhook URL
POST
https://services.tokenview.io/vipapi/monitor/stablecoin/setwebhookurl?apikey={apikey}
Parameters
- {apikey}: Get your activated apikey from the API system: https://services.tokenview.io
Request
curl -X POST -H 'content-type:text/plain' -d '{your_webhook_url}'
'https://services.tokenview.io/vipapi/monitor/stablecoin/setwebhookurl?apikey={apikey}'
Response
{
"code": 1,
"msg": "success",
"data": null
}
Response Description
{
"action": "ISSUE", // Value: MINT,ISSUE,DESTORY,REDEEM
"amount": "19000000",
"from": "0x5754284f345afc66a98fbb0a0afe71e0f007b949",
"fromAlias": "Tether Treasury",
"height": 12076299,
"network": "ETH:0xdac17f958d2ee523a2206206994597c13d831ec7",
"stableCoinName": "USDT",
"time": 1616251614,
"to": "0xa953550fdcd9d08fe362bc4a5f3561b26988833d",
"txid": "0x40562f0f3206d78a928c1a2faf751213eb5d693819cf72700a43261210ec876a"
}
Get webhook URL
GET
https://services.tokenview.io/vipapi/monitor/stablecoin/getwebhookurl?apikey={apikey}
Parameters
- {apikey}: Get your activated apikey from the API system: https://services.tokenview.io
Request
curl --location https://services.tokenview.io/vipapi/monitor/stablecoin/getwebhookurl?apikey={apikey}
Response
{
"code": 1,
"msg": "success",
"data": "https://9aa90255-9cde-453e-83a6-2916cd2c3725.mock.pstmn.io/webhook"
}
Get Tracked StableCoin List
GET
https://services.tokenview.io/vipapi/monitor/stablecoin/list?page={page}apikey={apikey}
Parameters
- {page}: page number
- {apikey}: Get your activated apikey from the API system: https://services.tokenview.io
Please specify the page.
Request
curl --location https://services.tokenview.io/vipapi/monitor/stablecoin/list?page=0&apikey={apikey}
Response
{
"code": 1,
"msg": "success",
"data": {
"page": 1,
"size": 7,
"total": 7,
"list": [
"busd",
"gusd",
"husd",
"pax",
"susd",
"tusd",
"usdc"
]
}
}
Add StableCoin
GET
https://services.tokenview.io/vipapi/monitor/stablecoin/add/{lowercase-coin-abbr}?apikey={apikey}
Parameters
- {lowercase-coin-abbr}: usdt, usdc, gusd,susd, tusd, pax, husd
- {apikey}: Get your activated apikey from the API system: https://services.tokenview.io
Note:
Support:USDT,USDC,GUSD,sUSD,TUSD,PAX,HUSD
Request
curl --location https://services.tokenview.io/vipapi/monitor/stablecoin/add/usdt?apikey={apikey}
Response
{
"code": 1,
"msg": "success",
"data": null
}
Delete StableCoin
GET
https://services.tokenview.io/vipapi/monitor/stablecoin/remove/{lowercase-coin-abbr}?apikey={apikey}
Parameters
- {lowercase-coin-abbr}: usdt, usdc, gusd,susd, tusd, pax, husd
- {apikey}: Get your activated apikey from the API system: https://services.tokenview.io
Request
curl --location https://services.tokenview.io/vipapi/monitor/stablecoin/remove/usdt?apikey={apikey}
Response
{
"code": 1,
"msg": "success",
"data": null
}
Get Webhook Record List
GET
https://services.tokenview.io/vipapi/monitor/stablecoin/webhookhistory/{lowercase-coin-abbr}?page={page}apikey={apikey}
Parameters
- {lowercase-coin-abbr}: usdt, usdc, gusd,susd, tusd, pax, husd
- {page}: page number
- {apikey}: Get your activated apikey from the API system: https://services.tokenview.io
Note:
- Specify the lowercase-coin-abbr and page
- Return all call records of a certain currency, and cannot specify the address for records.
- Each page returns a fixed 100 call records.
Request
curl --location https://services.tokenview.io/vipapi/monitor/stablecoin/webhookhistory/eth?page=1&apikey={apikey}
Response
{
"code": 1,
"msg": "success",
"data": {
"page": 1,
"size": 100,
"total": 3785,
"list": [
{
"coin": "ETH",
"url": "https://9aa90255-9cde-453e-83a6-2916cd2c3725.mock.pstmn.io/webhook",
"response": "ok",
"txid": "0xd832d6af2b89ad5f192578cdfe3c4a2102890f33407047162a794dcf36ab1f22",
"address": "0x5a0b54d5dc17e0aadc383d2db43b0a0d3e029c4c",
"confirmations": 0,
"tryTimes": 0,
"height": 10255999,
"statusCode": 200
},
{
"coin": "ETH",
"url": "https://9aa90255-9cde-453e-83a6-2916cd2c3725.mock.pstmn.io/webhook",
"response": "ok",
"txid": "0x16e36cca0bbe24743e82c250b01a1344af7b652efca53c078452f8f01772eb88",
"address": "0xab5c66752a9e8167967685f1450532fb96d5d24f",
"confirmations": 0,
"tryTimes": 0,
"height": 10254563,
"statusCode": 200
}
]
}
}